ListTileの特定行に対して、Widgetを重ねる方法。
この例では、行の右上に三角マークを付与している。
●コード
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Custom ListTile Example',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: ListTileWithTriangle(),
);
}
}
class ListTileWithTriangle extends StatelessWidget {
@override
Widget build(BuildContext context) {
final List<Map<String, dynamic>> data = [
{'title': 'Item 1', 'highlight': false},
{'title': 'Item 2', 'highlight': true},
{'title': 'Item 3', 'highlight': false},
{'title': 'Item 4', 'highlight': true},
];
return Scaffold(
appBar: AppBar(title: Text('Custom ListTile Example')),
body: ListView.builder(
itemCount: data.length,
itemBuilder: (context, index) {
final item = data[index];
return Stack(
children: [
ListTile(
title: Text(item['title']),
tileColor: item['highlight'] ? Colors.yellow[100] : Colors.white,
),
if (item['highlight'])
Positioned(
top: 0,
right: 0,
child: CustomPaint(
size: Size(30, 30), // 三角形のサイズ
painter: TrianglePainter(Colors.red),
),
),
],
);
},
),
);
}
}
class TrianglePainter extends CustomPainter {
final Color color;
TrianglePainter(this.color);
@override
void paint(Canvas canvas, Size size) {
final Paint paint = Paint()..color = color;
final Path path = Path()
..moveTo(size.width, 0) // 右上
..lineTo(size.width, size.height) // 右下
..lineTo(size.width - size.height,0 ) // 左下
..close(); // 三角形を閉じる
canvas.drawPath(path, paint);
}
@override
bool shouldRepaint(CustomPainter oldDelegate) => false;
}
●出力
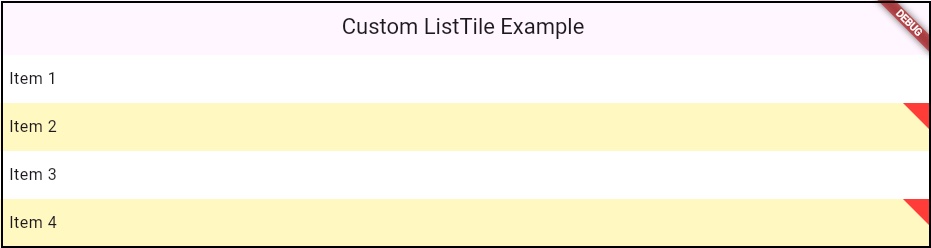